Welcome back to this series on building threat hunting tools. In this series, I will be showcasing a variety of threat hunting tools that you can use to hunt for threats, automate tedious processes, and extend to create your own toolkit!
The majority of these tools will be simple, with a focus on being easy to understand and implement. This is so that you, the reader, can learn from these tools and begin to develop your own. There will be no cookie-cutter tutorial on programming fundamentals like data types, control structures, etc., this series will focus on the practical implementation of scripting through small projects.
It is encouraged that you play with these scripts, figure out ways to break or extend them, and try to improve on their basic design to fit your needs. I find this the best way to learn any new programming language/concept and, certainly, the best way to derive value!
In this installment, we will look at creating EXEs (executables) from a Python file.
What is an Executable?
An executable is a computer program or a file that contains machine code instructions that can be executed directly by a computer’s operating system. If you are using a Windows machine, they’ll be familiar to you as the files on your computer that you double-click to run some task or operation. These files commonly end in a .exe
extension and range from simple scripts to entire games with complex graphics.
But why do we need executables when we can just run a Python script?
There are several reasons why you might want to create executables from your Python scripts:
- Ease of distribution: Python scripts require Python to be installed on the machine they are executed on. By creating a standalone program, from a Python file, you can easily share a program without the need for Python or any dependencies.
- Portability: Executables provide portability across different operating systems and environments. By converting a Python script into an executable, you can ensure that the program can run on systems that may not have Python installed or where the required Python version may be different.
- Protecting source code: Converting a Python file into an executable can help protect the source code of your script from being easily accessible or modified by others. This is particularly important if you have secrets (API keys, credentials, etc.) in your script.
- Improved performance: In some cases, converting a Python script into an executable can result in improved performance. By bundling the necessary Python interpreter and libraries with the executable you can eliminate the overhead of dynamically interpreting the code, leading to faster execution speed.
In this demonstration, we will be using the Python module py2exe to turn our Python scripts into executable programs. This module is able to convert Python scripts into standalone executables to run on Windows operating systems. It bundles your Python code, along with any required dependencies and resources, into a single executable file that can be distributed without the need for a Python installation.
Py2exe offers various configuration options that allow you to customize the behavior of the generated executable, such as specifying additional files to include, excluding certain modules, setting the icon for the executable, and more. Although we will stick to the basics in this demonstration you are encouraged to explore this other option.
The Problem
In a previous article, we created a basic Python script that used the Maltiverse API to check if an IP address was malicious right from the command line:
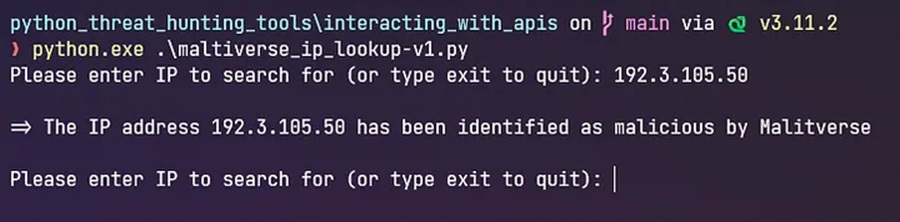
However, as previously discussed, Python is not installed on Windows machines by default. This means that we cannot run our Python threat hunting script on other Windows machines in its current version.
If we wanted to share this script with our colleagues or show it off to our friends, they would complain that it doesn’t work on their machine and we would be left looking like a fool. This is where we can use py2exe to save the day.
The Solution
Let’s see how we can use py2exe to turn our simple Python script into a standalone threat hunting tool that we can add to our arsenal.
Step #1: Install Py2exe
To begin with, we need to install the py2exe
module. This can be done using the pip package manager. In the command prompt enter
pip install py2exe
Step #2: Create a Setup Script
To run py2exe we need a setup script. This script specifies the configuration for py2exe to use when creating our executable file. The following is a basic setup script.
from py2exe import freeze
freeze(
console=[{'script': 'maltiverse_ip_lookup-v1.py'}],
options={'py2exe': {'bundle_files': 1, 'compressed': True}},
zipfile = None
)
This script:
- uses py2exe’s
freeze()
function to set parameters. - sets the script that is to be transformed into an executable (
maltiverse_ip_lookup-v1.py
). - defines several py2exe options to limit the number of files created and the size of the executable.
- stops py2exe from adding all files created to a ZIP file (the default behavior).
Step #3: Run Py2exe Using the Setup Script
Now we can run py2exe, in the command prompt, specifying the setup script we are using to define our configuration parameters.
python setup.py py2exe
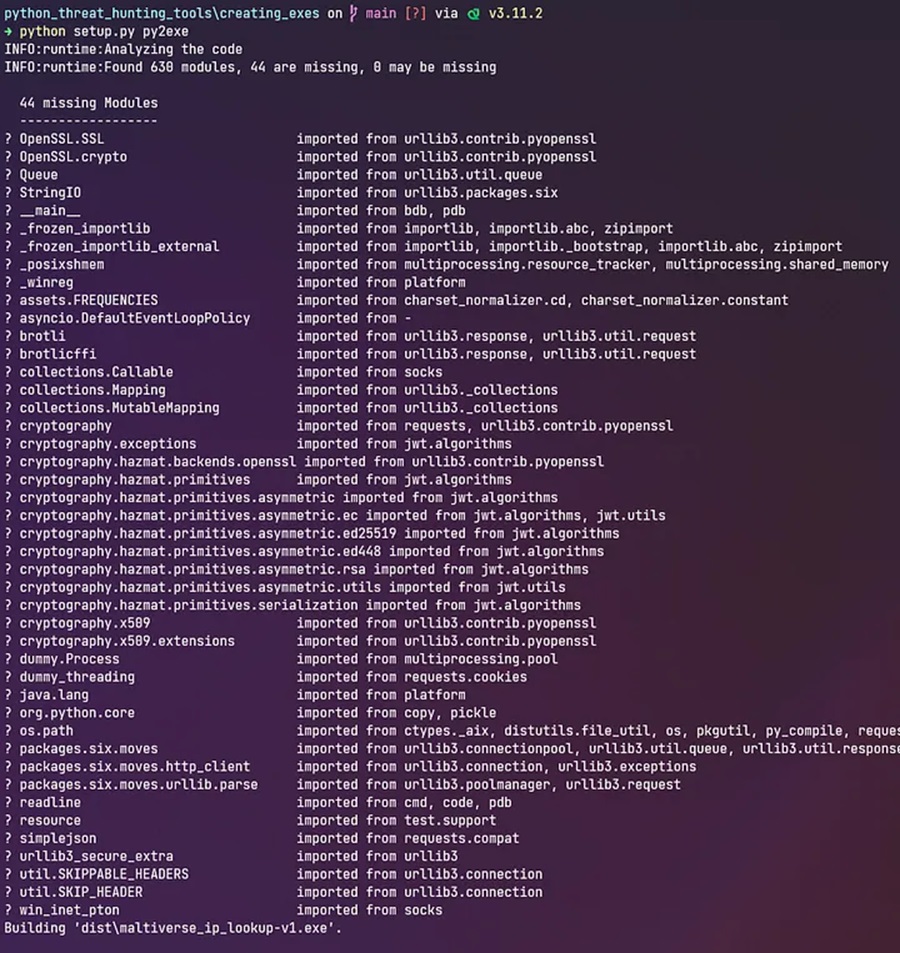
Step #4: Find the Executable
Py2exe will analyze our malicious IP checker script and find its dependencies (e.g. the ipaddress
and maltiverse
Python modules). It will bundle these together and produce an executable in a new dist directory. The generated files may include the main executable file, Python DLLs, required modules, and any additional files specified in the setup.py
script.
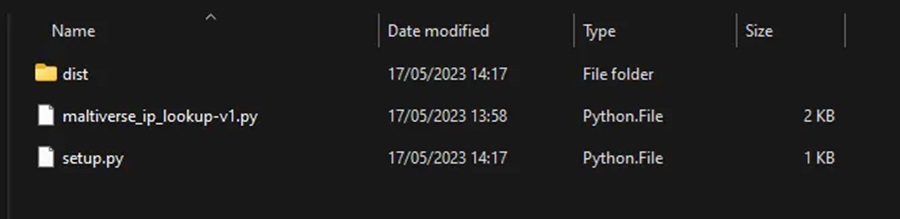
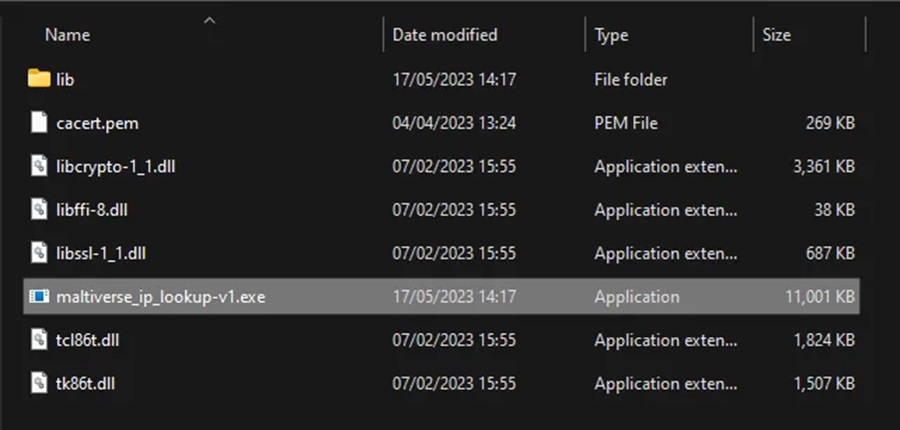
Step #5: Distributing our Threat Hunting Tool
With our executable created, we can now distribute it to our colleagues and friends. They will be able to run this tool on their systems and, hopefully, be impressed by our craftsmanship.
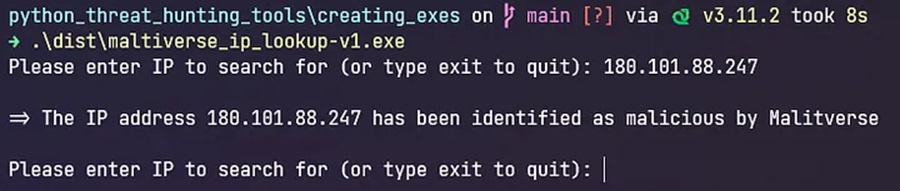
It’s important to note that py2exe may have some limitations and compatibility issues with certain libraries or modules. Make sure to check the py2exe documentation and consider testing your executable on different Windows environments to ensure proper functionality.
The full code for this demonstration can be found on GitHub in the creating_exes
directory.
I recommend that you expand on what was shown here. Read the documentation for py2exe and see what other fun configuration options you can specify. Then turn other Python scripts you have created into executables and share them around.
Next time in this series we will look at parsing CSV files so we can extract and use data stored within. Till then, happy hunting!
Discover more in the Python Threat Hunting Tools series!